情境
Toast是一個小型提示訊息的視窗,會顯示在視窗下方的一個小小訊息,不會妨礙使用者進行其他的操作,是一個很常見的工具。
完整程式碼
如果你需要完整程式碼,可以到 GitHub 上觀看或下載。
程式碼+說明
這邊會示範三種操作:
- 正常顯示訊息的 Toast
- 可調整位置的 Toast
- 客製化後的 Toast
一開始我們先設定三個按鈕,當我們按下去的時候,就可以跳出對應的 Toast。
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button android:id="@+id/normal_toast"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Normal Toast" />
<Button android:layout_below="@id/normal_toast"
android:id="@+id/normal_position_toast"
android:text="Position Toast"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<Button android:layout_below="@id/normal_position_toast"
android:id="@+id/custom_toast"
android:text="Position Toast"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</RelativeLayout>
如下圖所示。
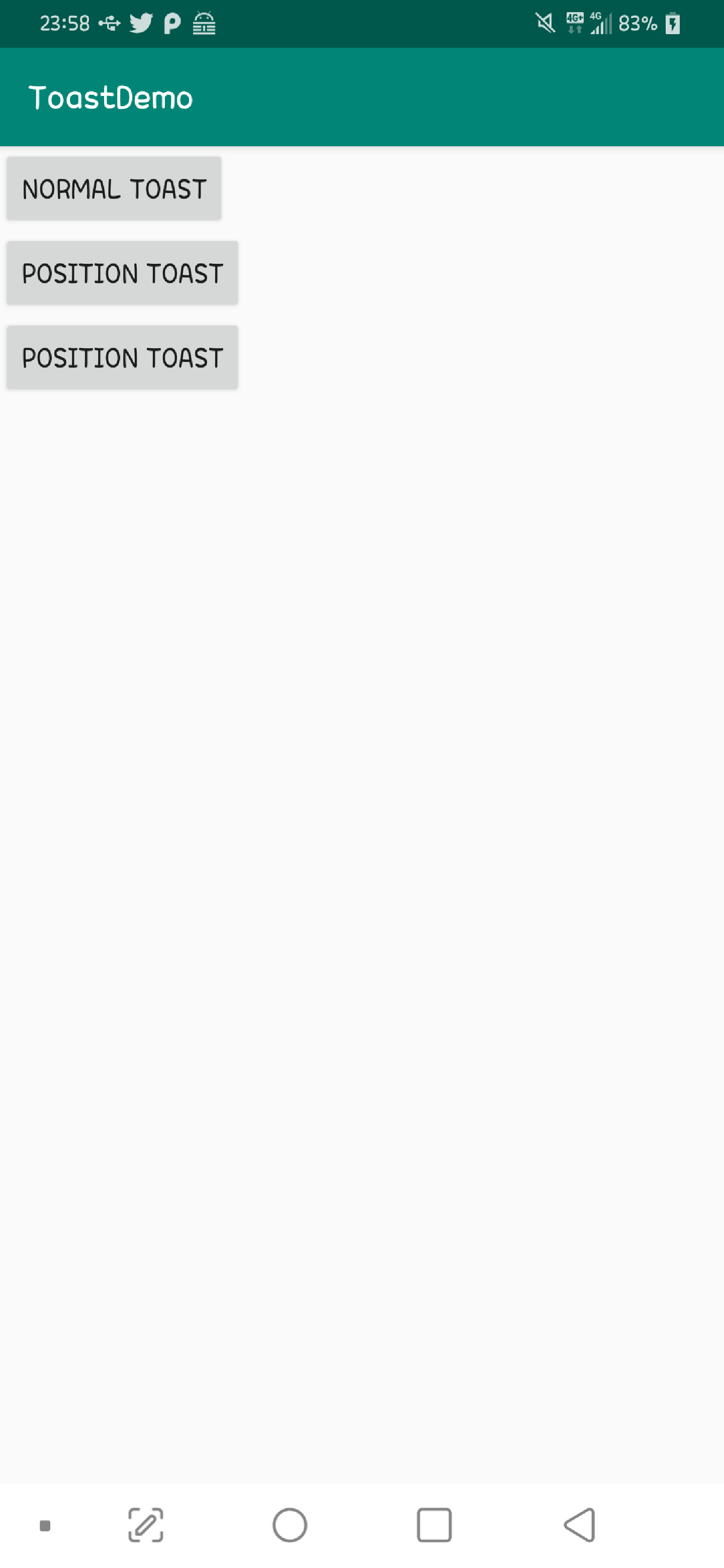
最基本的操作就是顯示一個訊息。
val context = applicationContext
val text = "Hello toast!"
val duration = Toast.LENGTH_SHORT
val toast = Toast.makeText(context, text, duration)
toast.show()
由上面的程式碼可以看到,單純顯示一個Hello Toast是多麼簡單,只需要告知它訊息跟顯示時間就可以直接使用了。
如下圖所示。
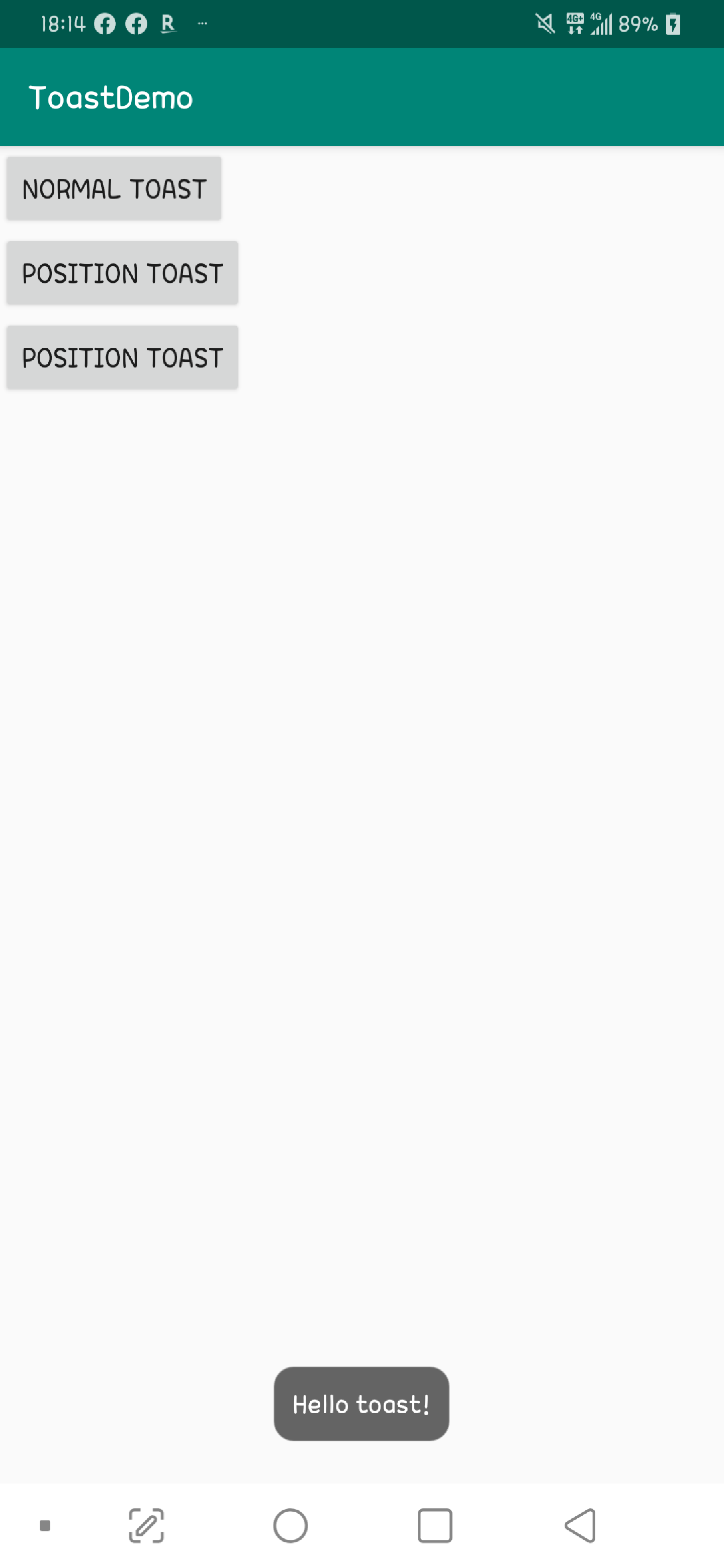
它也可以讓你選擇放置的位置,不一定非得要在下方。
val text = "Hello toast!"
val duration = Toast.LENGTH_SHORT
val toast = Toast.makeText(applicationContext, text, duration)
toast.setGravity(Gravity.CENTER_VERTICAL, 0, 0)
toast.show()
如下圖所示。
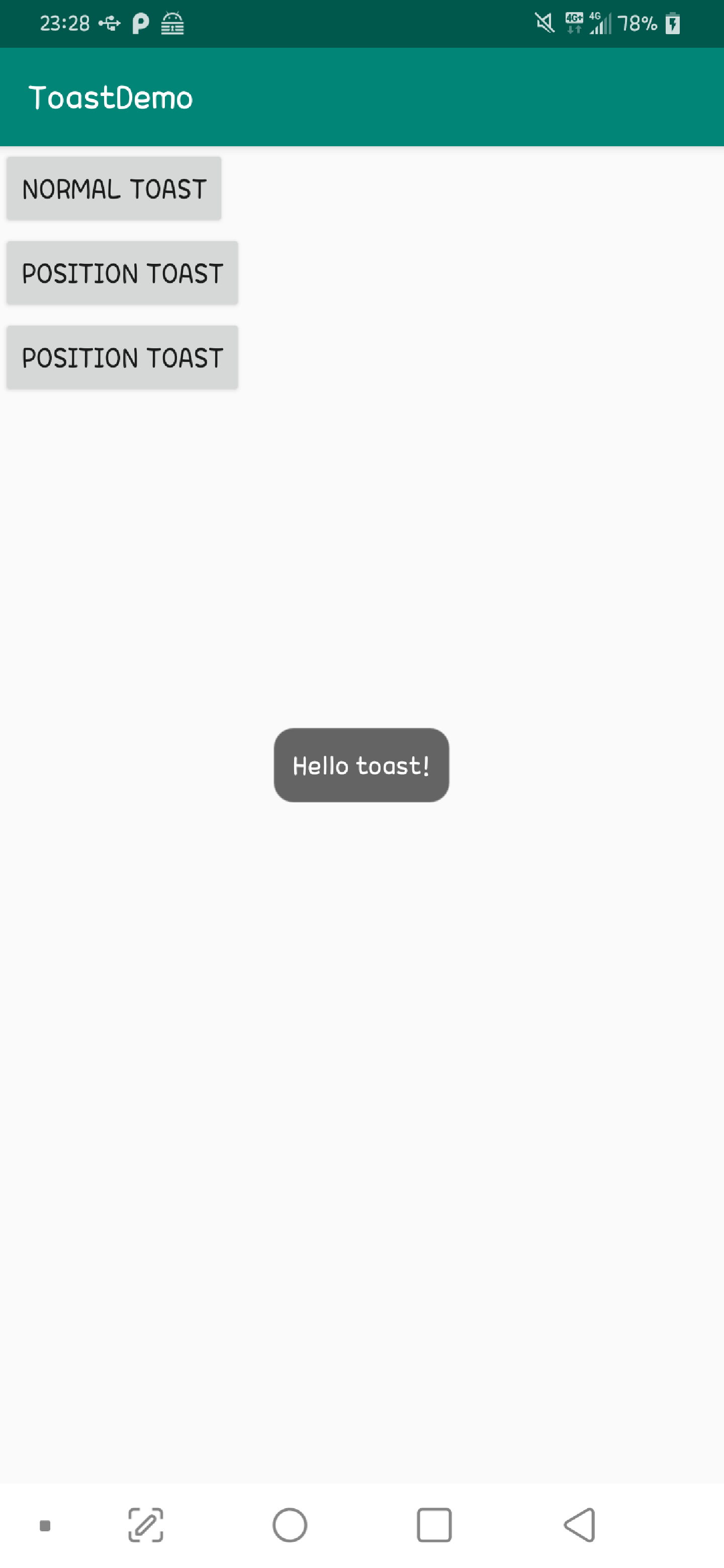
你甚至可以透過setView的方法來設定自己布局的畫面。
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/custom_toast_container"
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="8dp"
android:background="@android:color/holo_orange_light">
<ImageView android:src="@drawable/droid"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginRight="8dp" />
<TextView android:id="@+id/text_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textColor="@android:color/black" />
</LinearLayout>
xml 設定好了以後,就可以顯示在自己自訂的Toast內。
val v = layoutInflater.inflate(R.layout.custom_toast, custom_toast_container, false)
v.text_view.text = getString(R.string.msg)
with(Toast(this)) {
duration = Toast.LENGTH_LONG
view = v
show()
}
如下圖所示。
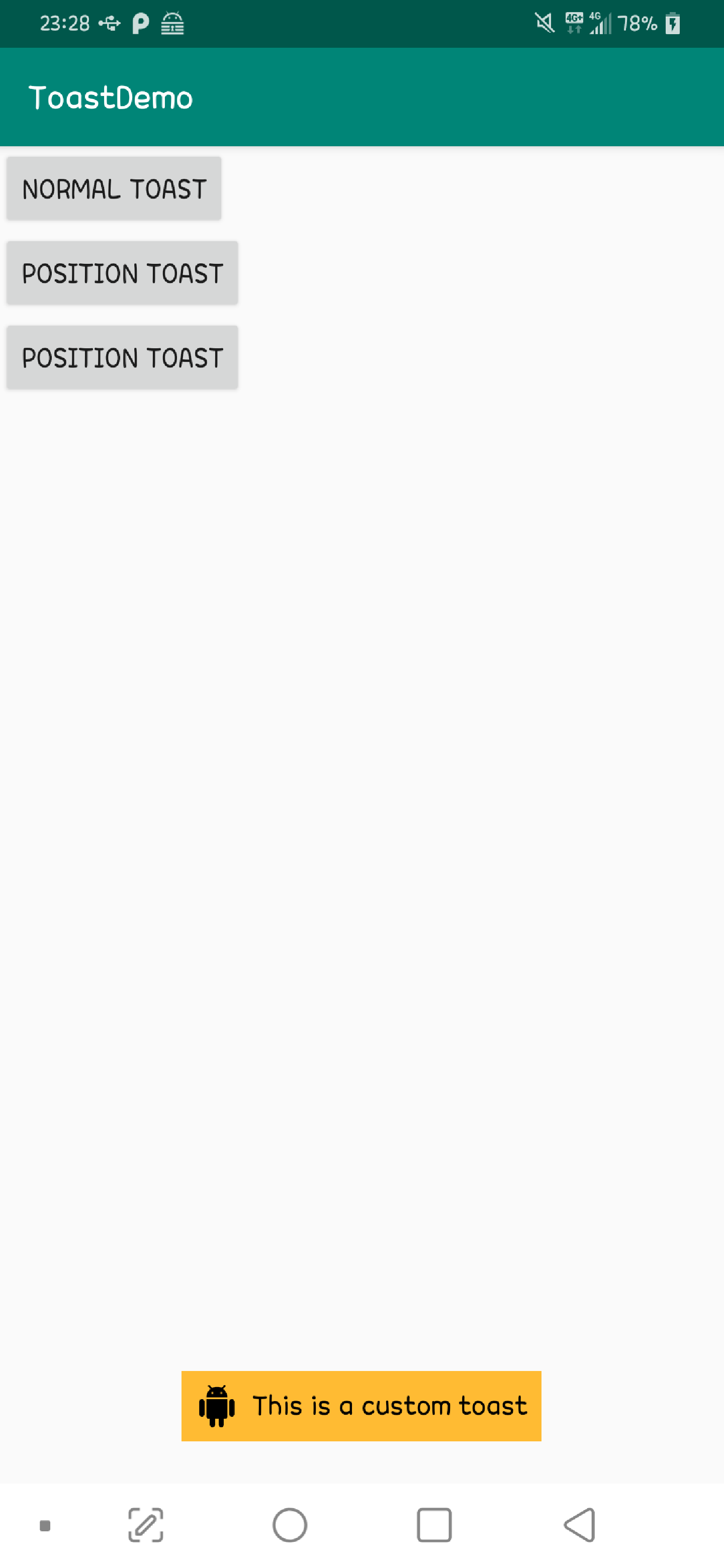
這樣就是一個簡單的Toast操作了。