情境
如果你想要宣告一個 Fragment 並且以全版面呈現,應該怎麼做?
完整程式碼
程式碼說明
一開始畫面呈現是由 Activity 啟動,所以在畫面上我們就塞了一個 Button,透過這個 Button 按下去的事件,來顯示我們的全版面的 DialogFragment。
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
btn.setOnClickListener {
val demoDialogFragment = DemoDialogFragment()
demoDialogFragment.show(supportFragmentManager, "")
}
}
}

接著來看 DialogFragment 的內容怎麼處理?
class DemoDialogFragment : androidx.fragment.app.DialogFragment() {
override fun onCreate(savedInstanceState: Bundle?) {
val dialog = super.onCreateDialog(savedInstanceState)
val window = dialog.window
window?.requestFeature(Window.FEATURE_NO_TITLE)
window?.setLayout(WindowManager.LayoutParams.MATCH_PARENT, WindowManager.LayoutParams.MATCH_PARENT)
setStyle(DialogFragment.STYLE_NORMAL, android.R.style.Theme_Light_NoTitleBar_Fullscreen)
super.onCreate(savedInstanceState)
}
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?): View? {
val v = inflater.inflate(R.layout.demo_fragment, container)
return v
}
}
可以看到在 DialogFragment 一開始要先宣告 Dialog 的形狀。
val dialog = super.onCreateDialog(savedInstanceState)
val window = dialog.window
window?.requestFeature(Window.FEATURE_NO_TITLE)
window?.setLayout(WindowManager.LayoutParams.MATCH_PARENT, WindowManager.LayoutParams.MATCH_PARENT)
setStyle(DialogFragment.STYLE_NORMAL, android.R.style.Theme_Light_NoTitleBar_Fullscreen)
DialogFragment 宣告的 layout 放在 R.layout.demo_fragment 裡面,裡面放入一個簡單的 TextView 並且顯示文字資訊。
- demo_fragment.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:text="這是全版 DialogFragment"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
app:layout_constraintTop_toTopOf="parent" app:layout_constraintEnd_toEndOf="parent"
android:layout_marginEnd="8dp" app:layout_constraintStart_toStartOf="parent"
android:layout_marginStart="8dp" android:layout_marginBottom="8dp"
app:layout_constraintBottom_toBottomOf="parent"/>
</androidx.constraintlayout.widget.ConstraintLayout>
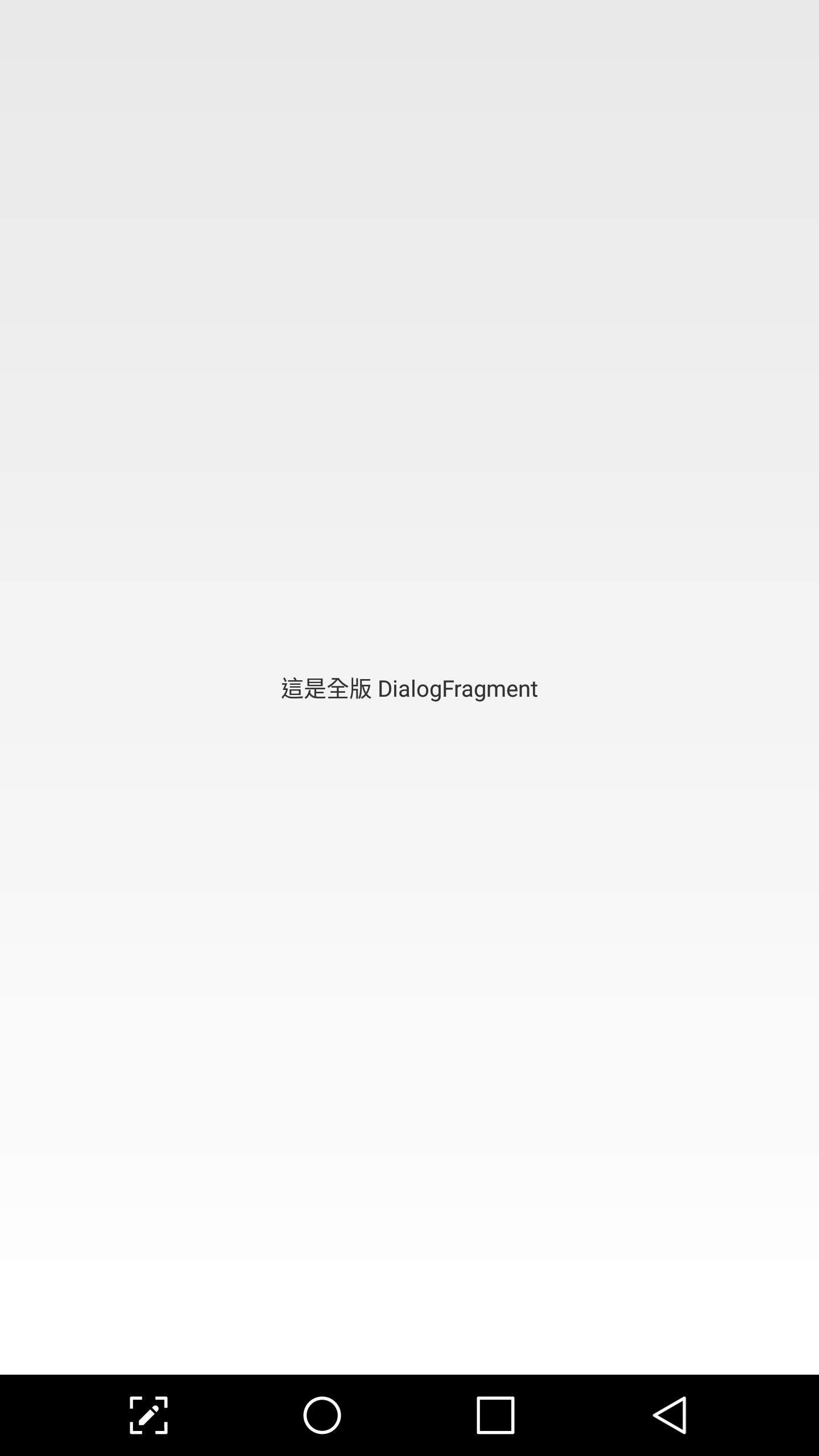
這樣就是一個簡單全版面的 DialogFragment 範例了。